I tried to build my website using Next.js instead of Nuxt.js.
Create the Next.js application
Create the template with the following command.
$ yarn create next-app sample-app --example with-tailwindcss with-tailwindcss-app
Design the website
Create the website design using Figma. It is not required but you easily write code so I recommend to use the prototyping tool.
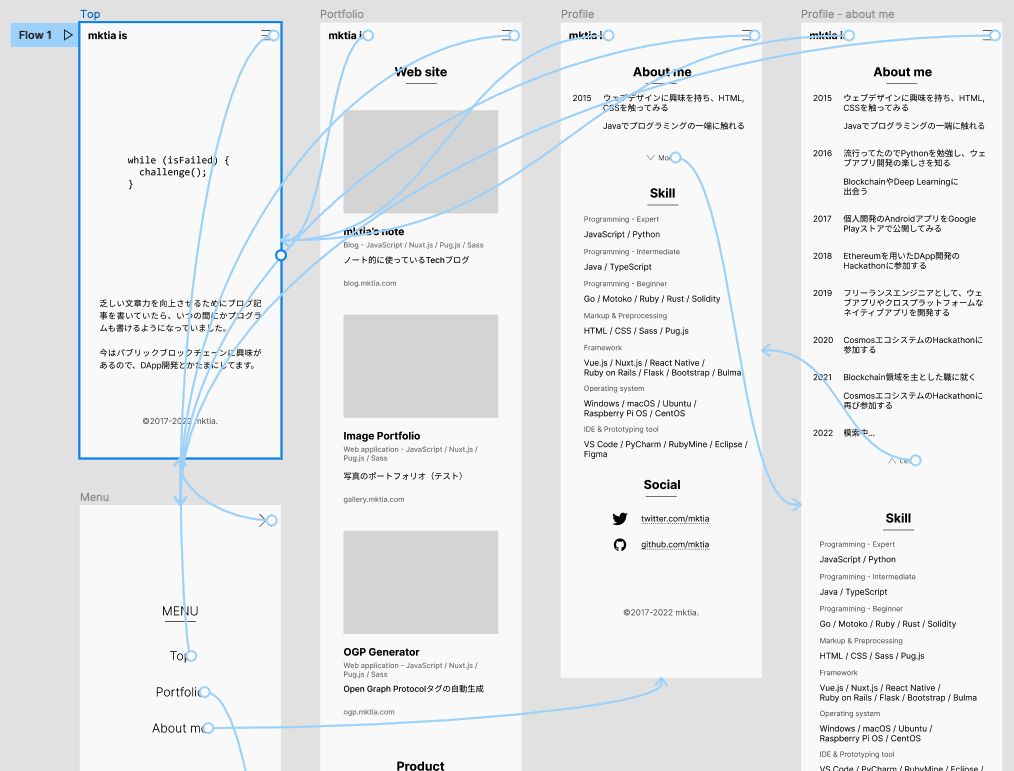
Create the component
React provides two ways to declare components: as classes or as functions.
Page
TSX files are automatically routed when they are prepared in the pages directory, which is provided by default.
import type { NextPage } from "next"
import Layout from "../components/layout"
const Home: NextPage = () => {
return (
<Layout>
<div>
<h2>index page</h2>
<p>This is the top page of this site.</p>
</div>
</Layout>
)
}
export default Home
Layout
Elements of the page are cut out and introduced as components.
import Head from "next/head"
import Footer from "./footer"
import Header from "./header"
import infoData from "../contents/info.json"
type Props = {
children?: React.ReactNode
title?: string
description?: string
}
const Layout = ({ children, title, description }: Props) => {
return (
<>
<Head>
<title>{title}</title>
<meta
name="description"
content={description || ""}
/>
</Head>
<Header />
<main className="container mx-auto px-4">{children}</main>
<Footer />
</>
)
}
export default Layout
The common elements for each page are created as a Layout
, which can be implemented by calling this component on each page. If you would like to pass arguments to a component, you can specify parameters to the function, as in Props
above.
Icon
Install the icon package.
$ npm i react-icons --save
Easily use an icon with the icon name tag.
import { FaBeer } from 'react-icons/fa';
const Example = () => {
return <h3> Lets go for a <FaBeer />? </h3>
}
export default Example
The icon names refer to the following page.
Reference: React Icons
Prepare the release
Implement the tags using Google Analytics and the Open Graph Protocol.
Deploy to server
In this time, I used Vercel. I deployed to Vercel via GitHub repository using GitHub Actions (CI/CD tools).